API design guide
API first approach or why API’s are important?
Today there is a growing need of sharing a large amount of data between various organizations, departments, devices, and common people.
A key tool for meeting these needs are most definitely API’s. API stands for Application Programming Interface, it is a door or a window in a software program. It acts as a robust and scalable data serving center, nothing more or less than that.
API first approach is an approach in which developer first build API and on top of that API web application is built. Furthermore, mobile applications are also built on top of the same API. By building on top of API with developers in mind, the company reduces down development time and frees developers of writing redundant code.
Trends are showing that most of IT companies are using this approach and most of the web applications are being converted to web API’s. There are also modern concepts like IoT (Internet of things) which is basically internetworking of physical devices, vehicles, buildings and household appliances such as refrigerator or stove.
With that in mind, it is obvious that API’s are future of web development and it is really important to standardize the way of making API’s.
RESTful API’s
Today there is no firmly defined standard for writing API’s, but there are some styling guidelines. The most popular and widely used architecture style is REST (Representation state transfer). REST principles rely on:
- Client-server communication
Client-server architecture has a very distinct separation of concerns, with migrating most of the business login from server to client. The server remains only as data serving center, with aim to be fast and reliable.
- Stateless
Server must be able to completely understand the client request without using any server context or server session state. It follows that all state must be kept on the client.
- Uniform Interface
All components must interact through a single uniform interface. Because all component interaction occurs via this interface, interaction with different services is very simple. The interface is the same. This also means that implementation changes can be made in isolation. Such changes will not affect fundamental component interaction because uniform interface is always unchanged.
The tradeoff for all of this flexibility is a lack of strongly set standards. REST really describes the method by which the data is transferred, but implementers have been left mostly on their own to figure out how this data should look.
Richardson maturity model
Richardson maturity model is a way to grade your API according to the constrains of REST. The better API adheres to these constrains, the higher its score is.
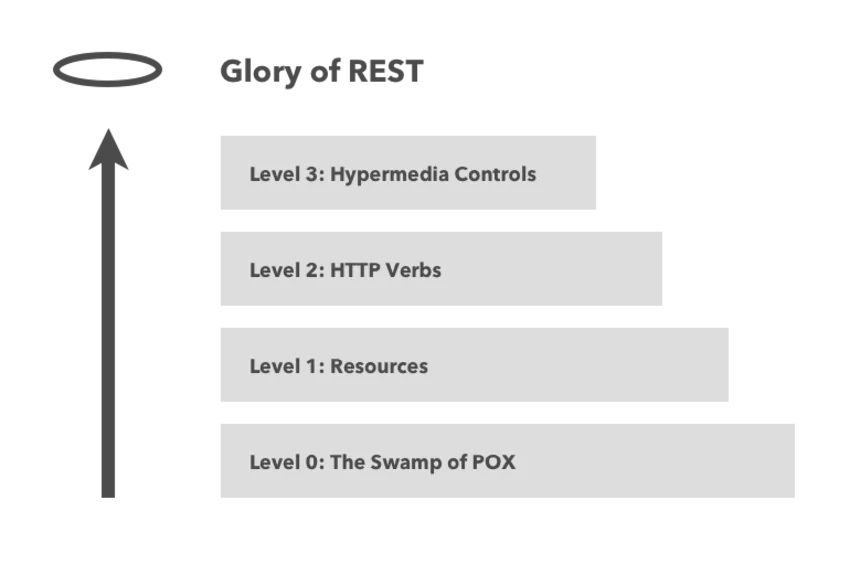
Richardson maturity model schema
- LEVEL 0: Swamp of POX
The starting point for the maturity model is to use HTTP purely as a transport system for remote interactions, but without using any of the mechanisms of the web. That is, it tunnels requests and responses through its protocol without using the protocol to indicate application state. It will use only one endpoint and only one kind of method (on this level usually POST)
- LEVEL 1: Resources
On this level goal is to introduce resources. So, rather than making all request to a singular service endpoint, we are now talking to individual resources. Resource is usually a data center in a web application or a model in an MVC pattern. This level uses multiple URI’s (endpoints) where every URI is an entry point to a specific resource. Instead of going through http://example.com/articles it is possible to distinguish between http://example.com/article/1 and http://example.com/article/2. This level still uses only one single method like POST
- LEVEL 2: HTTP verbs
This level standardizes the use of HTTP verbs to perform actions. This level indicates that API should use the protocol properties in order to deal with scalability and failures.
Basic HTTP verbs that are mostly used are GET and POST. HTTP defines GET as a safe operation that doesn’t make any significant changes to the state of anything. This allows us to invoke GET’s safely any number of times in any order and get the same result each time. On the other hand, POST method does changes state. Roughly we can say that POST methods are used to send some information to the server and write them to the database (change database state), and GET is used to retrieve data without making any changes to server state, even if we are sending some data parameters along with GET.
Other widely used HTTP verbs include PUT, PATCH, DELETE. PUT and PATCH are used for updating resource, only difference is that PUT updates whole resource while PATCH is used for partial updates. PUT is considered idempotent and safe because it does not change the state of server (it does not create new resource) while PATCH is not considered idempotent by default.
DELETE verb is used for delete operations. DELETE is also considered as idempotent operation.
Besides HTTP verbs other novelty on this level includes usage of status codes as a controller of a client workflow. If a GET request was successful response status code should be 200 (OK), if there was some error status code should be set accordingly (500) so client app can display error message or any other action necesary.
- LEVEL 3: Hypermedia controls (HATEOAS)
Example of hypermedia links
The point of hypermedia controls is that they tell us what we can do next, and the URI of the resource we need to manipulate to do it. Rather than is having to know where to post our appointment request, the hypermedia controls in the response tell us how to do it.
One benefit is that it allows the server to change its URI scheme without breaking clients. Similarly, it helps client developers explore the protocol. Links give client developers a hint as to what may be possible next.
Conclusion
API’s are the future of web development, the future that is approaching at really fast speed. Some standardization is needed more than ever. As a developer, I believe that Richardson maturity model is a way to go to make your API’s more standardized and more RESTfull.